Basic Concepts of Reactjs Previous Article learning with Doubtly’s ReactJS Introduction
Basic Concepts
Getting started with React involves understanding some foundational concepts. Let’s break them down in simple terms:
1. React ES6
You might have heard about ES6, it’s basically the latest version of JavaScript, bringing in some cool new features and syntax enhancements. React loves to use these because they make coding more efficient and readable.
So, when you’re coding in React, you’ll often see stuff like classes, arrow functions, and destructuring assignments, which are all part of ES6. They’re not essential for React, but they make your code look sleek and modern.
// Example ES6 syntax in React component
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
message: "Hello, World!"
};
}
render() {
return <div>{this.state.message}</div>;
}
}
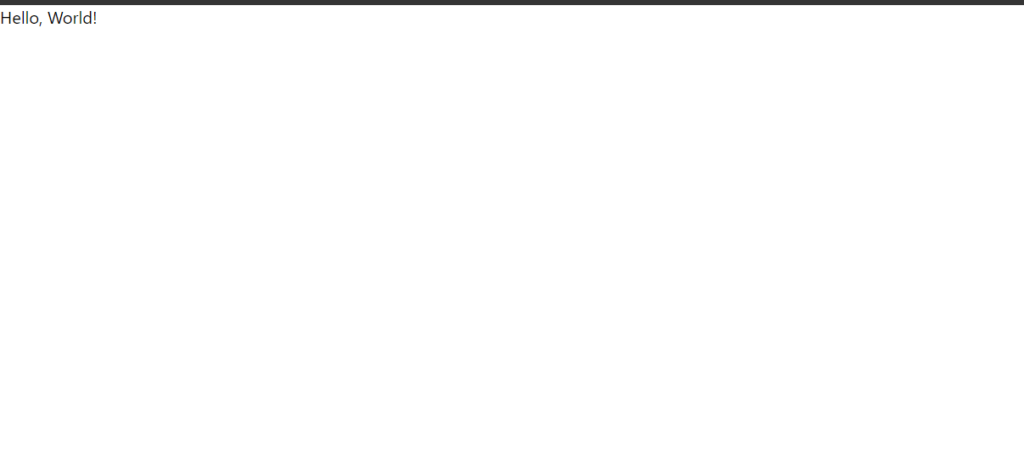
2. React Render HTML
Now, let’s talk about how React gets stuff on the screen. You know how you use document.createElement()
in vanilla JavaScript to create DOM elements? Well, in React, you use something called ReactDOM.render()
.
This is like the magician’s wand that makes your React elements appear on the webpage. You tell it what you want to render and where you want to render it, and bam! There it is.
// Example of rendering HTML element with ReactDOM.render()
const element = <h1>Hello, React!</h1>;
ReactDOM.render(element, document.getElementById('root'));
3. React JSX
Alright, imagine you’re writing your HTML code, and suddenly you need to insert some JavaScript logic. Traditionally, you’d have to break out of HTML and jump into JavaScript, right?
Well, JSX saves you from that hassle. It’s like a special language that lets you write HTML and JavaScript together. It looks like HTML, smells like HTML, but it’s actually JavaScript under the hood.
// Example of JSX syntax in React component
class MyComponent extends React.Component {
render() {
return <div>Hello, JSX!</div>;
}
}
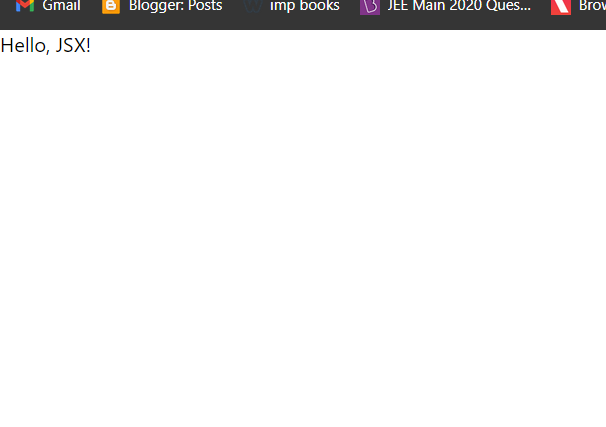
So, when you see tags in your React code that look like HTML but have JavaScript inside them, that’s JSX doing its magic!
Understanding these basics will give you a solid start in your React journey. ES6 makes your code fancier, ReactDOM.render()
puts your stuff on the screen, and JSX lets you write HTML and JavaScript in perfect harmony. Happy coding!