create a accordion with html css and javascript
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
body {
font-family: 'Arial', sans-serif;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
}
.accordion {
width: 300px;
border: 1px solid #ccc;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.accordion-section {
border-bottom: 1px solid #ccc;
}
.accordion-header {
padding: 10px;
cursor: pointer;
background-color: #f5f5f5;
user-select: none;
}
.accordion-body {
padding: 10px;
display: none;
}
</style>
</head>
<body>
<div class="accordion">
<div class="accordion-section">
<div class="accordion-header" onclick="toggleSection(1)">Section 1</div>
<div class="accordion-body" id="section1">This is the content for Section 1.</div>
</div>
<div class="accordion-section">
<div class="accordion-header" onclick="toggleSection(2)">Section 2</div>
<div class="accordion-body" id="section2">This is the content for Section 2.</div>
</div>
<div class="accordion-section">
<div class="accordion-header" onclick="toggleSection(3)">Section 3</div>
<div class="accordion-body" id="section3">This is the content for Section 3.</div>
</div>
</div>
<script>
function toggleSection(sectionNumber) {
var section = document.getElementById('section' + sectionNumber);
section.style.display = (section.style.display === 'block') ? 'none' : 'block';
// Hide other sections
for (var i = 1; i <= 3; i++) {
if (i !== sectionNumber) {
document.getElementById('section' + i).style.display = 'none';
}
}
}
</script>
</body>
</html>
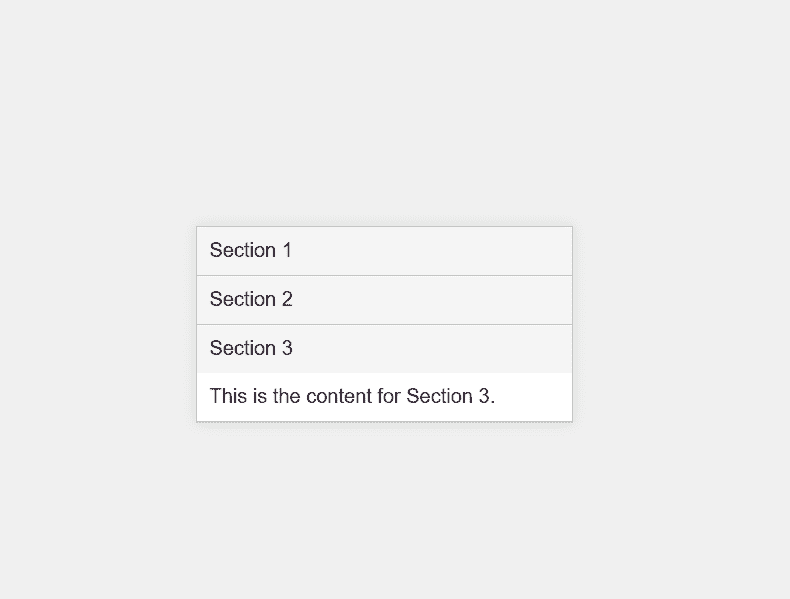